Hello Lucid Devs and community. I'm trying to get access to the data fields attached to a LucidSpark Card that was created using your Jira data connector/integration. I spoke with you all recently and you had suggested I leverage ElementProxy, but I’m having trouble getting it to work. Would greatly appreciate any help about where I’m going wrong. If you have any example code for this scenario that would be helpful as well.
My 'extension.ts' file (stripped down to focus on just getting data fields):
import {
EditorClient,
Menu,
MenuType,
Viewport,
CardBlockProxy,
DataProxy,
ElementProxy,
CollectionProxy,
} from "lucid-extension-sdk";
const client = new EditorClient();
const menu = new Menu(client);
const viewport = new Viewport(client);
const data = new DataProxy(client);
async function listDataFieldsForSelectedElement(elementId: string) {
const elementProxy = new ElementProxy(elementId, client);
let fieldsSet = new Set();
for (const referenceKey of elementProxy.referenceKeys.values()) {
const dataItemProxy = referenceKey.getItem();
console.log("DataItemProxy:", dataItemProxy);
if (!dataItemProxy) {
console.error("DataItemProxy not found for referenceKey:", referenceKey);
continue;
}
const collectionProxy = dataItemProxy.collection;
console.log("CollectionProxy:", collectionProxy);
// Check if the CollectionProxy object has the fields you expect
if (collectionProxy && "getFields" in collectionProxy) {
const fields = collectionProxy.getFields();
console.log("Fields in Collection:", fields);
fields.forEach((field) => fieldsSet.add(field));
} else {
console.error(
"CollectionProxy is undefined or does not have the getFields method for dataItemProxy:",
dataItemProxy
);
}
}
return Array.from(fieldsSet);
}
client.registerAction("readCardProperties", () => {
const selectedItems = viewport.getSelectedItems(true);
if (!selectedItems || selectedItems.length === 0) {
client.alert("No items are selected.");
return;
}
for (const item of selectedItems) {
if (item instanceof CardBlockProxy) {
try {
listDataFieldsForSelectedElement(item.id)
.then((refFieldsAsString) => {
const content = `Ref Fields: ${refFieldsAsString.join(", ")}`;
console.log("Content:", content);
})
.catch((error) => {
// Handle any errors that occur during the fetch
console.error("Error reading card properties:", error);
});
} catch (error) {
console.error("Error reading card properties:", error.message);
}
} else {
console.error("Selected item is not a CardBlockProxy instance.");
}
}
});
client.registerAction("itemsSelected", () => {
const items = viewport.getSelectedItems(true);
return items && items.length > 0;
});
menu.addMenuItem({
label: "Read Card Properties",
action: "readCardProperties",
menuType: MenuType.Context,
visibleAction: "itemsSelected",
});
Screen capture of browser console:
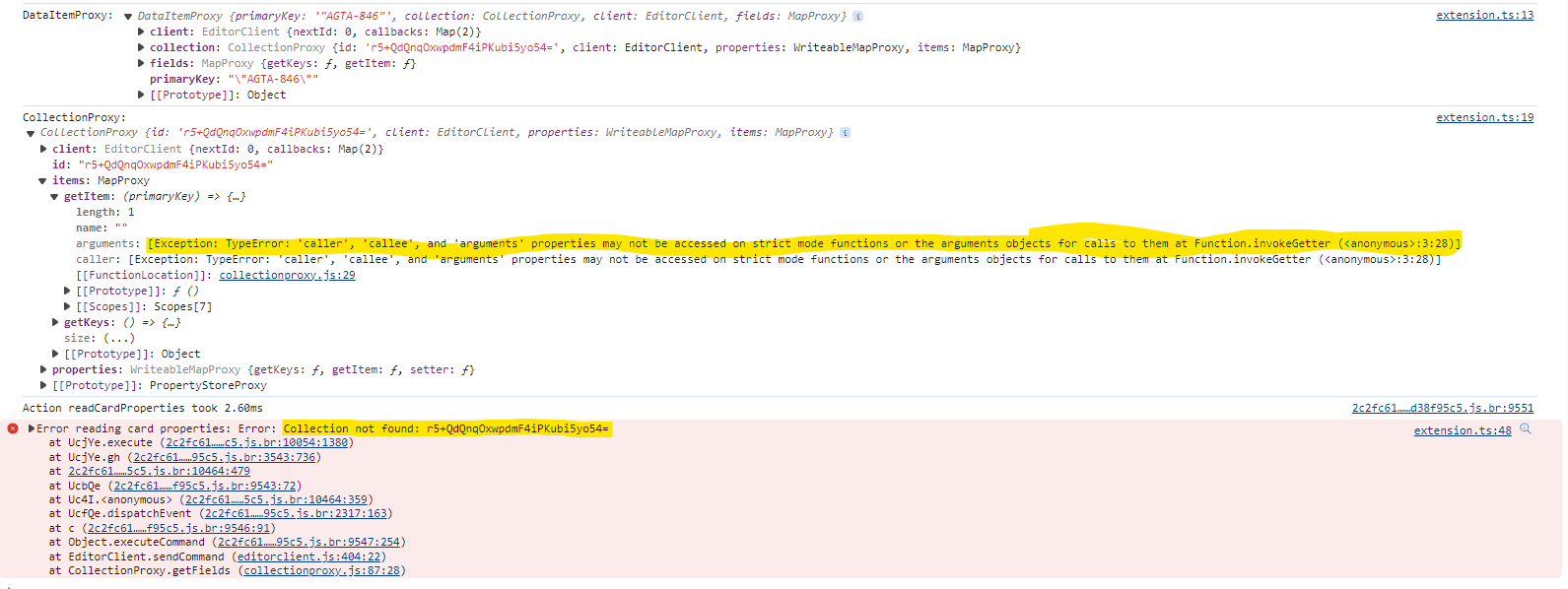
Copy of console log output when action called in case you want to see it:
EditorClient, fields: MapProxy}client: EditorClient {nextId: 0, callbacks: Map(3)}collection: CollectionProxyclient: EditorClient {nextId: 0, callbacks: Map(3)}id: "r5+QdQnqOxwpdmF4iPKubi5yo54="items: MapProxygetItem: (primaryKey) => {…}length: 1name: ""arguments: aException: TypeError: 'caller', 'callee', and 'arguments' properties may not be accessed on strict mode functions or the arguments objects for calls to them
at Function.invokeGetter (<anonymous>:3:28)]caller: :Exception: TypeError: 'caller', 'callee', and 'arguments' properties may not be accessed on strict mode functions or the arguments objects for calls to them
at Function.invokeGetter (<anonymous>:3:28)]moFunctionLocation]]: collectionproxy.js:29tiPrototype]]: ƒ ()otScopes]]: ScopesS7]getKeys: () => {…}size: (...); Prototype]]: Objectproperties: WriteableMapProxy {getKeys: ƒ, getItem: ƒ, setter: ƒ}tIPrototype]]: PropertyStoreProxyfields: MapProxy {getKeys: ƒ, getItem: ƒ}primaryKey: "\"AGTA-846\""riPrototype]]: Object
extension.ts:39 CollectionProxy: CollectionProxy {id: 'r5+QdQnqOxwpdmF4iPKubi5yo54=', client: EditorClient, properties: WriteableMapProxy, items: MapProxy}client: EditorClient {nextId: 0, callbacks: Map(3)}id: "r5+QdQnqOxwpdmF4iPKubi5yo54="items: MapProxy {getKeys: ƒ, getItem: ƒ}properties: WriteableMapProxy {getKeys: ƒ, getItem: ƒ, setter: ƒ}KePrototype]]: PropertyStoreProxyconstructor: class CollectionProxygetBranchedFrom: ƒ getBranchedFrom()getFields: ƒ getFields()length: 0name: "getFields"arguments: eException: TypeError: 'caller', 'callee', and 'arguments' properties may not be accessed on strict mode functions or the arguments objects for calls to them
at Function.invokeGetter (<anonymous>:3:28)]caller: tException: TypeError: 'caller', 'callee', and 'arguments' properties may not be accessed on strict mode functions or the arguments objects for calls to them
at Function.invokeGetter (<anonymous>:3:28)]n.FunctionLocation]]: collectionproxy.js:86unPrototype]]: ƒ ()coScopes]]: Scopes:7]getLocalChanges: ƒ getLocalChanges()getName: ƒ getName()getSchema: ƒ getSchema()getSyncCollectionId: ƒ getSyncCollectionId()patchItems: ƒ patchItems(patch)CoPrototype]]: Object
2c2fc614ad2208c1a1f0bcb45e551fca796817167ded9dc88b07efd38f95c5.js.br:9551 Action readCardProperties took 2.80ms
extension.ts:78 Error reading card properties: Error: Collection not found: r5+QdQnqOxwpdmF4iPKubi5yo54=